MORAI Simulator Control API
MSC API provides a non-UI-based control method of the Simulator using communications API. To use the Simulator, the user is required to input various settings and parameters prior to testing. The MSC API allows the user to facilitate configuring the settings and enables the automation of Simulator tests based on given scenarios.
Setup
Download Example Code
Download API Documentation
Python Version
The example code has been written and tested in Python 3.7.3
.
How to Run
Run api.py
after configuring user-specific settings in params.txt
within the example code.
File description
api.py
This file executes the Simulator based on information inputted in params.txt.
params.txt
params.txt
includes parameter information used by the .py file.The following must be configured: IP, port, user_id, user_pw, version, Map/Vehicle.
receive user IP: IP for receiving information (data, command results) from Launcher / Simulator
receive user port: Port for receiving information from Launcher / Simulator
request DST IP: IP for command transmission to Launcher / Simulator
request DST port: Port for command transmission to Launcher / Simulator
user_id: User ID
user_pw: User password
version: Version of Simulator
map: Map to play in Simulator (case sensitive)
vehicle: Vehicle to play in Simulator (case sensitive)
network_file: Name of network file to configure (Launcher Data → SaveFile→ Network)
sensor_file: Name of sensor file to configure (Launcher Data → SaveFile→ Sensor)
scenario_file: Name of scenario file to configure (Launcher Data → SaveFile→ Scenario)
Parameter defined in params.txt
LinkConnection.txt
SaveFile\LinkConnection.txt
inside Launcher folderLinkConnection.txt contains IP and Port information for Launcher / Simulator
IP and Port have to be configured as follows.
Receive Host IP: IP of Launcher / Simulator for receiving commands via API
Receive Host PORT: Port of Launcher / Simulator for receiving commands via API
Request Destination IP: Destination IP for sending information from Launcher / Simulator
Request Destination PORT: Destination Port for sending information from Launcher / Simulator
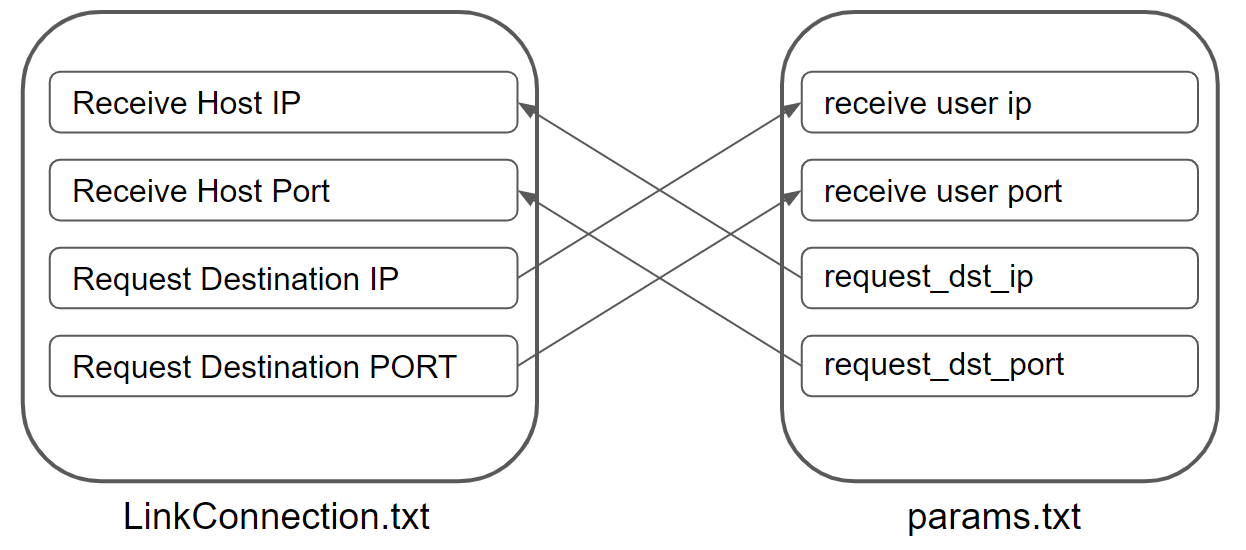
Command Description
A Controller class method,
commander()
from controller.py, is used inlauncher_start_api.py
.Commands used are pre-defined in the
Command_list
Class fromdefine.py
.
##launcher_start_api.py
if self.controller.is_befor_login():
self.controller.commander(Command.LOGIN)#Login명령
if self.controller.is_after_login() or self.controller.is_after_sim_quit_to_launcher(): # is_after_sim_quit_to_launcher : Simulator에서 quit 명령 후 Launcher 복귀 상태 확인
self.controller.commander(Command.SELECT_VER)#version select명령
if self.controller.is_not_find_version(): #version_error
break
if self.controller.is_can_execute_sim():
self.controller.commander(Command.EXECUTE_SIM) #Simulator 실행 명령
self.controller.watting_execute()
if self.controller.is_sim_not_install():
self.controller.commander(Command.INSTALL_SIM) #Simulator 설치 명령
if self.controller.is_sim_lobby():
self.controller.commander(Command.MAP_VEHICLE_SELECT)#시뮬레이션/옵션 변경 실행 명령
self.controller.watting_loading()
if self.controller.is_sim_playing():
self.controller.commander(Command.NET_SETTING) #Network setting
self.controller.commander(Command.SEN_SETTING) #Sensor setting
self.controller.commander(Command.SCEN_SETTING) #Scenraio setting
##define.py
class Command_list(Enum):
#Launcher Command
LOGIN = (Platform.LUANCHER, cmd.LOGIN , user_id+'/'+user_pw)
SELECT_VER = (Platform.LUANCHER, cmd.SELECT_VER , version)
INSTALL_SIM = (Platform.LUANCHER, cmd.INSTALL_SIM , "")
EXECUTE_SIM = (Platform.LUANCHER, cmd.EXECUTE_SIM , "")
QUIT_LAUNCHER = (Platform.LUANCHER, cmd.QUIT_LAUNCHER , "")
LOGOUT = (Platform.LUANCHER, cmd.LOGOUT , "")
#Simulator Command
MAP_VEHICLE_SELECT = (Platform.SIMULATOR, cmd.MAP_VEHICLE_SELECT , map+'/'+vehicle)
SIM_PAUSE = (Platform.SIMULATOR, cmd.SIM_PAUSE , "")
SIM_PLAY = (Platform.SIMULATOR, cmd.SIM_PLAY , "")
NET_SETTING = (Platform.SIMULATOR, cmd.NET_SETTING , network_file)
NET_SAVE = (Platform.SIMULATOR, cmd.NET_SAVE , )
SEN_SETTING = (Platform.SIMULATOR, cmd.SEN_SETTING , sensor_file)
SEN_SAVE = (Platform.SIMULATOR, cmd.SEN_SAVE , "")
SCEN_SETTING = (Platform.SIMULATOR, cmd.SCEN_SETTING , scenario_file+"/62")
SCEN_SAVE = (Platform.SIMULATOR, cmd.SCEN_SAVE , "")
QUIT_SIM = (Platform.SIMULATOR, cmd.QUIT_SIM , "")
Flow Chart for Example Code
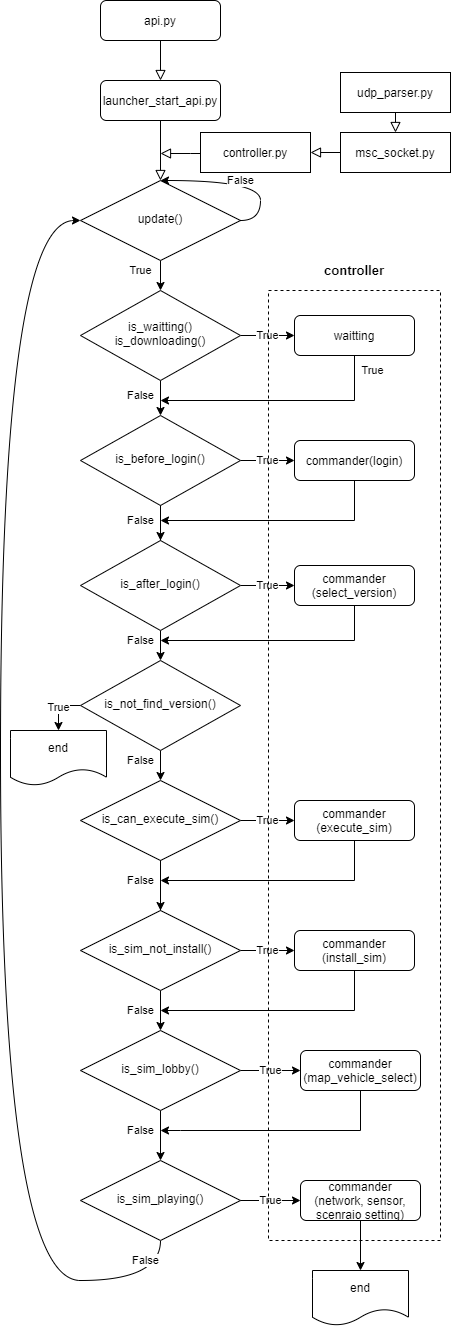
Refer to the link below for details
Check API availability
API availability can be checked in the indicators placed in the upper left-hand corner of Launcher / lower left-hand corner of Simulator
Green: MORAI Simulator Control API is available
Red: MORAI Simulator Control API is unavailable (Check IP and Port numbers)
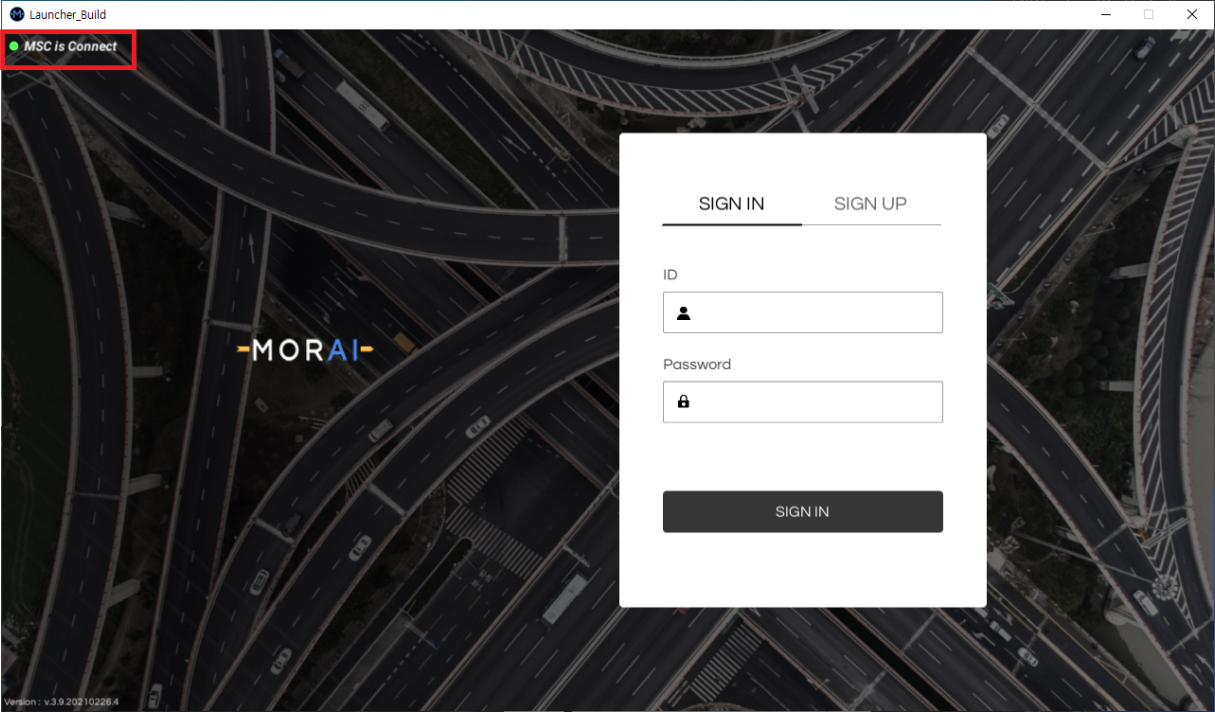
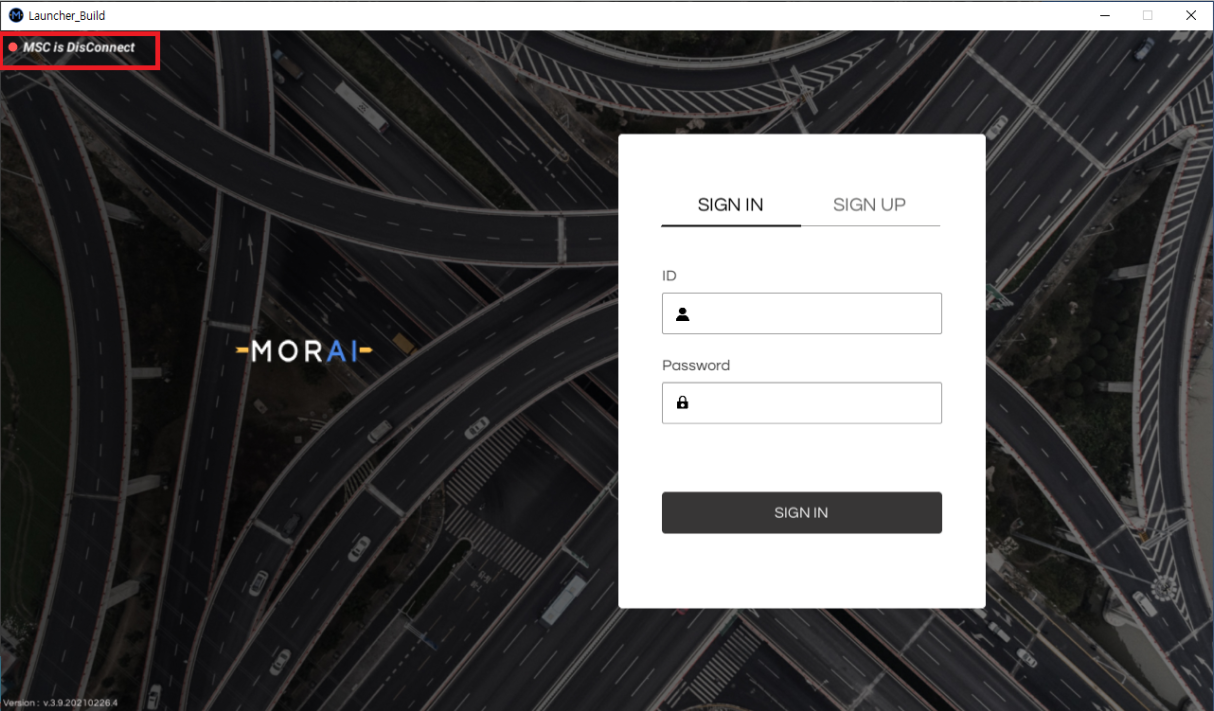
API is available (MSC is connected)
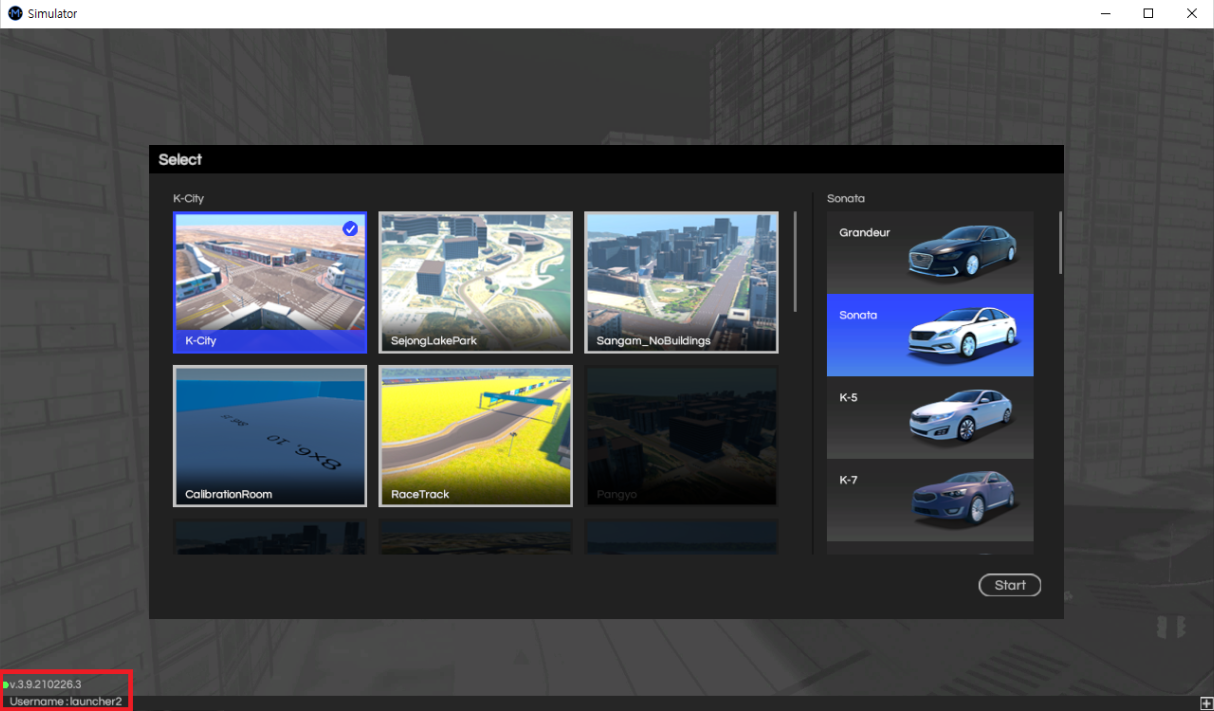
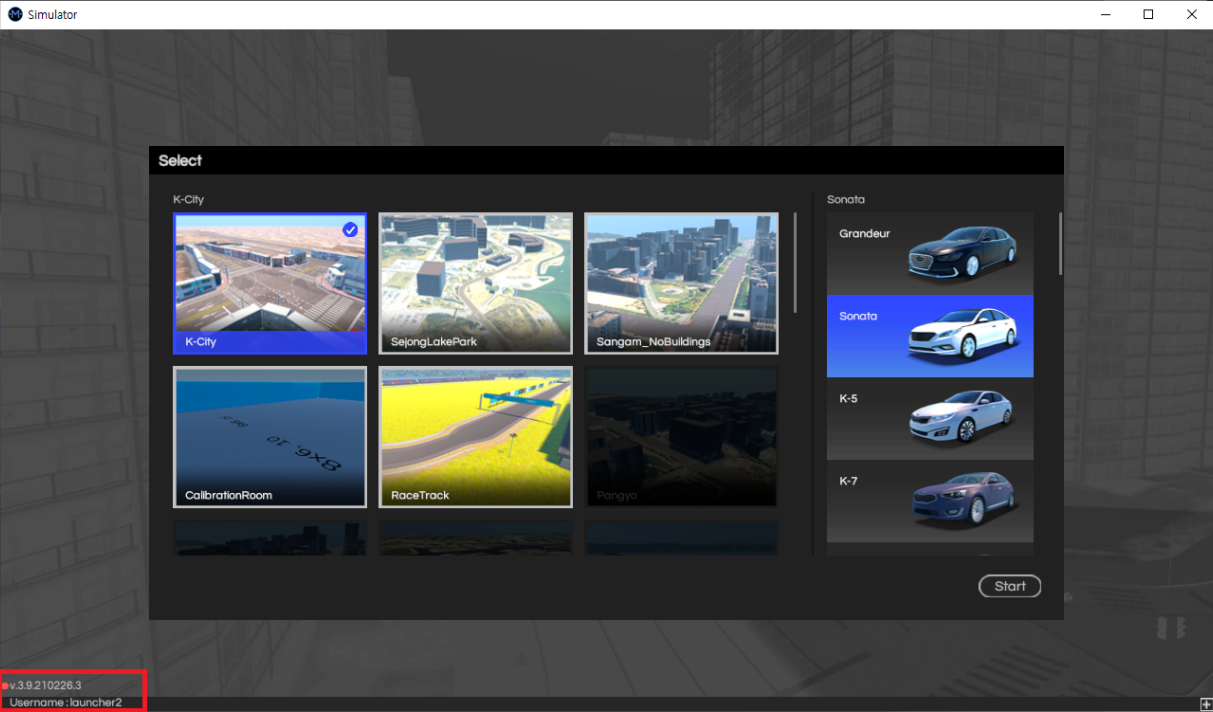
API is unavailable (MSC is disconnected)
Example Use Case
The example video shows how to set up and run api.py
.